Use the Entire Screen
Published by @SoNiceInfo at 6/24/2020
There are 2 ways to use the entire screen.
- Expand
frame
maxWidth and maxHeight ofVStack
to infinity - Apply
.edgesIgnoringSafeArea(.all)
toZStack
Expand frame
of VStack
Setting .infinity
of frame
, the view is expanded to the entire screen remaining the safe area.
Here is the example code applied infinity to frame. backgound()
is colored to make it easier to understand.
//
// ContentView.swift
//
import SwiftUI
struct ContentView: View {
@State var name: String = ""
var body: some View {
VStack {
Spacer()
Text("Result: ") + Text(name)
TextField("Placeholder", text: $name)
.padding()
.border(Color.green, width: CGFloat(2))
Spacer()
}
// ここが大事
.frame(minWidth: 0, maxWidth: .infinity, minHeight: 0, maxHeight: .infinity, alignment: .topLeading)
.background(Color.yellow)
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
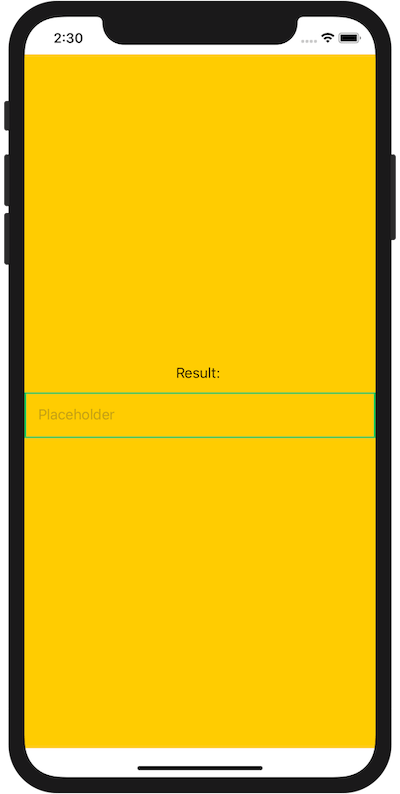
Apply .edgesIgnoringSafeArea(.all)
to ZStack
Applying .edgesIgnoringSafeArea(.all)
, the view is expanded ignoring the safe area.
In ZStack
, the first View is at the bottom, and the second View is at the top.
Make the background yellow to make the effect easier to see.
//
// ContentView.swift
//
import SwiftUI
struct ContentView: View {
@State var name: String = ""
var body: some View {
ZStack {
Color.yellow
.opacity(0.4)
// ここが大事
.edgesIgnoringSafeArea(.all)
VStack {
Text("Result: ") + Text(name)
TextField("Placeholder", text: $name)
.padding()
.border(Color.green, width: CGFloat(2))
}
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
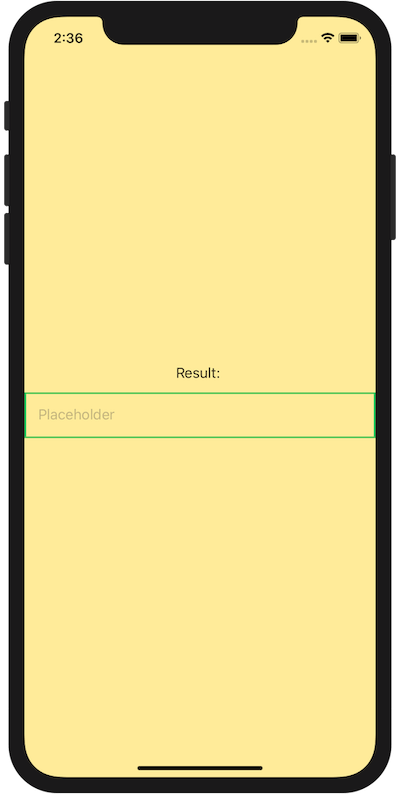
References
Make a VStack fill the width of the screen in SwiftUI - Stack OverflowHow to expand SwiftUI views to span across entire width or height of screen - Simple Swift Guide