Input/Display Text
Published by @SoNiceInfo at 6/24/2020
Use Text()
to display text.
Use TextField()
and SecureField()
to input.
The basic usage is as follows.
In the input, we need to pass the bind variable, so we use the $
symbol.
Please see Understanding Property Wrappers to handle data.
//
// ContentView.swift
//
import SwiftUI
struct ContentView: View {
@State var text: String = ""
var body: some View {
Text("SwiftUIへの道")
TextField("Placeholder", text: $text)
SecureField("", text: $text)
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
Change Style
There are modifiers for changing the text style by default.forgroundColor()
(Change the text color)background()
(Change the background. You can also insert an image for the background.) bold()
(make it bold) . italic()
(to italicize). strikethrough()
(add strikethrough line)underline()
(underline). tracking()
(changes the character spacing)
.
//
// ContentView.swift
//
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
Text("SwiftUIへの道")
Text("SwiftUIへの道")
.foregroundColor(.orange)
Text("SwiftUIへの道")
.background(Color.purple)
Text("SwiftUIへの道")
.bold()
Text("SwiftUIへの道")
.italic()
Text("SwiftUIへの道")
.strikethrough(true, color: .black)
Text("SwiftUIへの道")
.underline(true, color: .red)
Text("SwiftUIへの道")
.baselineOffset(CGFloat(30))
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
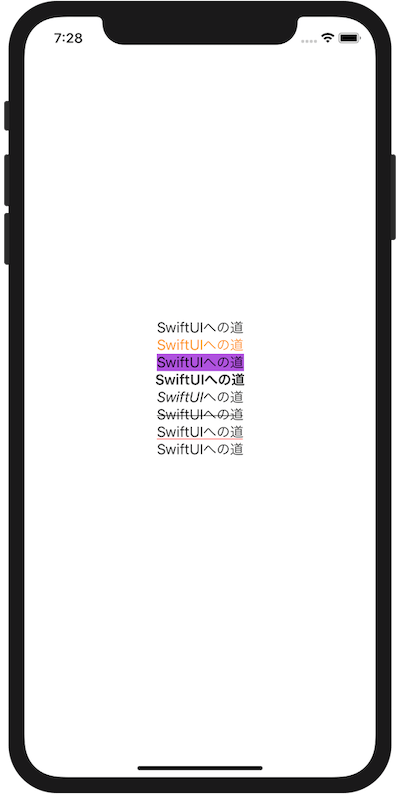
Change Font
To change the font, use font()
and fontWeight()
.
font()
has eight default formats that can be changed by default.largeTitle
title
headline
body
and so on.
Use custom()
to set the font and size freely.
Use system()
to set the size, weight and design.
Use fontWeight
to specify the weight of the font.
The following example uses the above to change the text to various fonts.
//
// ContentView.swift
//
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
Text("これはデフォルトです")
Text("これは大タイトルです")
.font(.largeTitle)
Text("これはタイトルです")
.font(.title)
Text("これは見出しです。")
.font(.headline)
Text("これは本文です")
.font(.body)
Text("太さを指定します")
.fontWeight(Font.Weight.ultraLight)
Text("種類、サイズを指定します")
.font(.custom("Futura-Med", size: 24))
Text("サイズ、太さ、種類を指定します")
.font(.system(size: 18, weight: .heavy, design: .serif))
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
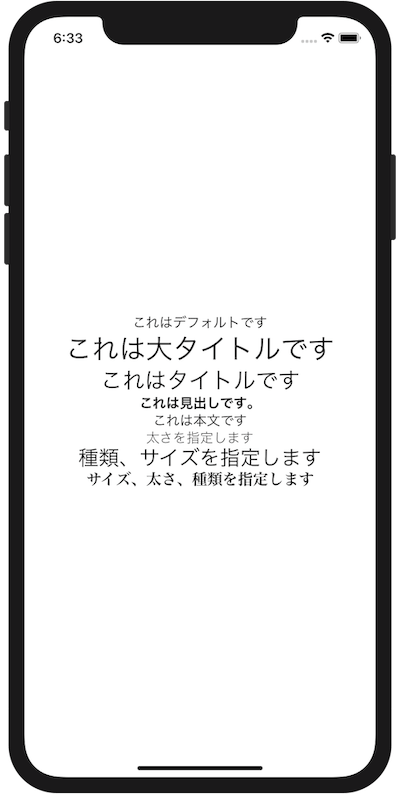
Input Text
Use TextField
to input text in SwiftUI.
Use SecureField
to enter text you don't want to display, such as a password.
The following example stores the value of the user's input in the text
variable.
You can use @State to let the SwiftUI manage changes to the text
variable.
Then you can pass the Bind value to TextField
and SecureField
to save the user-entered value to the variable text
.
In the demo image, by inputting value in the upper TextField
, the result is reflected in the lower TextField
, SecureField
and Text
.
//
// ContentView.swift
//
import SwiftUI
struct ContentView: View {
@State var text: String = ""
var body: some View {
VStack {
// Input
TextField("Placeholder", text: $text)
TextField("Placeholder", text: $text)
.foregroundColor(.blue)
.textFieldStyle(RoundedBorderTextFieldStyle())
SecureField("", text: $text)
.textFieldStyle(RoundedBorderTextFieldStyle())
// Output
Text("↓")
Text(text)
.border(Color.red, width: 2.0)
}.padding()
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
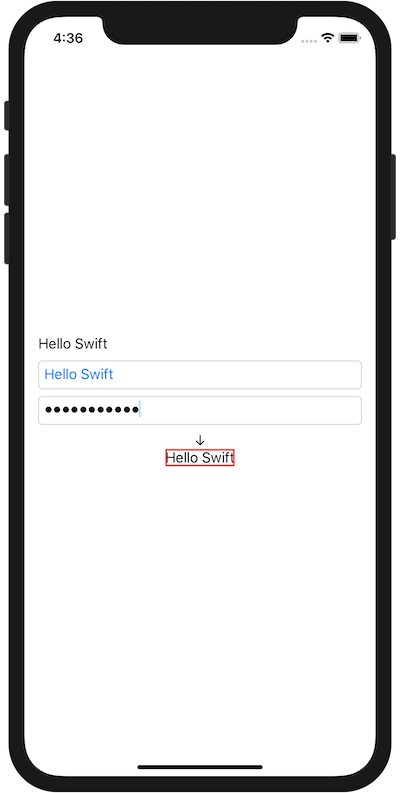
Please see Sharing Data over Views more about handling input data.