Create Instagram Home Screen
Published by @SoNiceInfo at 6/24/2020
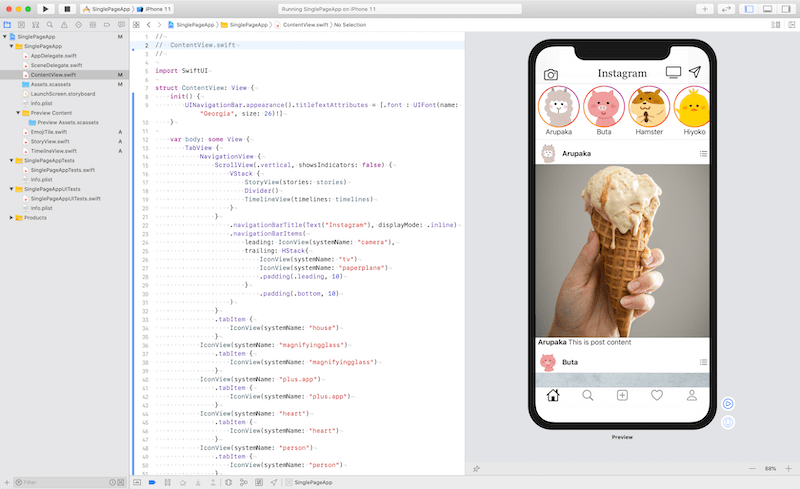
Elements to create a home screen
Title bar and tabs (blue frame)
ContentView.swift
Story (orange frame)
StoryView.swift
Timeline (green box)
TimelineView.swift
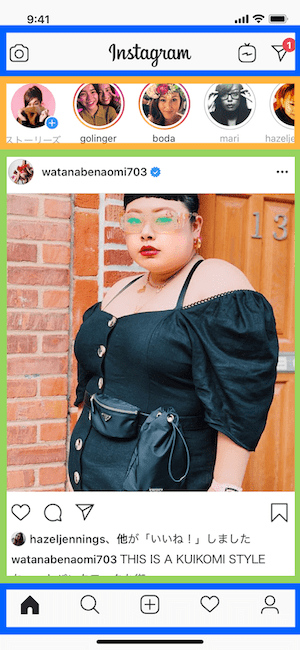
Title bar and tabs (blue frame) - ContentView.swift
Create title bar and tabs with combination of NavigationView
and TabView
.
The font of title bar can't change SwiftUI, use UINavigationBar.appearance()
in Initializer.
//
// ContentView.swift
//
import SwiftUI
struct ContentView: View {
init() {
UINavigationBar.appearance().titleTextAttributes = [.font : UIFont(name: "Georgia", size: 26)!]
}
var body: some View {
TabView {
NavigationView {
Text("Here for Stories and Timelines")
.navigationBarTitle(Text("Instagram"), displayMode: .inline)
.navigationBarItems(
leading: IconView(systemName: "camera"),
trailing: HStack{
IconView(systemName: "tv")
IconView(systemName: "paperplane")
.padding(.leading, 10)
}
.padding(.bottom, 10)
)
}
.tabItem {
IconView(systemName: "house")
}
IconView(systemName: "magnifyingglass")
.tabItem {
IconView(systemName: "magnifyingglass")
}
IconView(systemName: "plus.app")
.tabItem {
IconView(systemName: "plus.app")
}
IconView(systemName: "heart")
.tabItem {
IconView(systemName: "heart")
}
IconView(systemName: "person")
.tabItem {
IconView(systemName: "person")
}
}
.edgesIgnoringSafeArea(.top) // Not needed after Xcode11.4
.accentColor(.black)
}
}
struct IconView: View {
var systemName: String
var body: some View {
Image(systemName: systemName)
.font(.title)
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
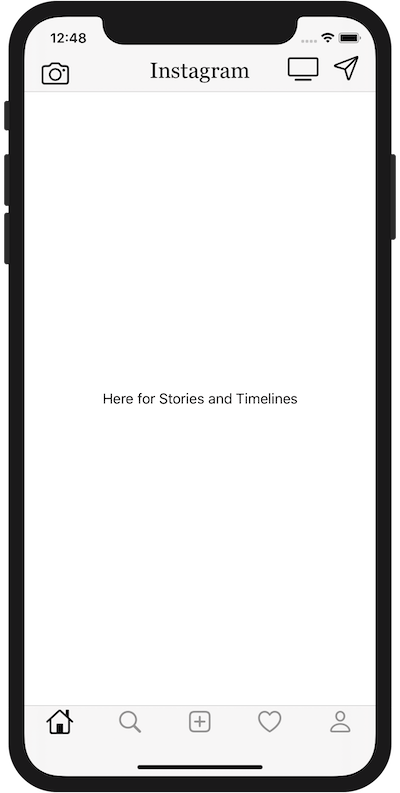
Story (orange frame) - StoryView.swift
Create a template with struct Story
.
Create a mock with let stories: [Story]
.
Use ScrollView(.horizonal)
to make scrollable left and right.
Use ForEach
to display contents of stories
one by one.
You must not forget to make story's frame gradation with Instagram color.
//
// StoryView.swift
//
import SwiftUI
struct Story {
let id: Int
let name: String
let image: String
}
let stories: [Story] = [
Story(id: 0, name: "Arupaka", image: "animal_arupaka"),
Story(id: 1, name: "Buta", image: "animal_buta"),
Story(id: 2, name: "Hamster", image: "animal_hamster"),
Story(id: 3, name: "Hiyoko", image: "animal_hiyoko"),
Story(id: 4, name: "Inu", image: "animal_inu")
]
struct StoryView: View {
let stories: [Story]
var body: some View {
ScrollView(.horizontal, showsIndicators: false) {
HStack() {
ForEach(stories, id: \.id) { (story) in
VStack(spacing: 0) {
ZStack {
Image(story.image)
.resizable()
.overlay(
Circle().stroke(LinearGradient(gradient: Gradient(colors: [.yellow, .red, .purple]), startPoint: .bottomLeading, endPoint: .topTrailing), lineWidth: 5))
.frame(width: 100, height: 100)
.clipShape(Circle())
}
Text(story.name)
}
}
}
.padding(.top, 5)
.padding(.leading, 5)
}
}
}
struct StoryView_Previews: PreviewProvider {
static var previews: some View {
StoryView(stories: stories)
}
}
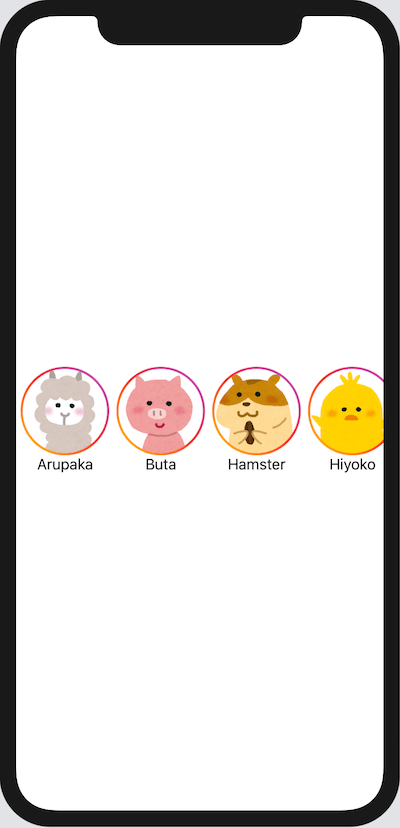
Timeline (green box) - TimelineView.swift
Create a template with struct Timeline
.
Create a mock with let timelines: [Timeline]
.
Make image square with screen width by UIScreen.main.bounds.width
.
Build elements with VStack
and HStack
.
//
// TimelineView.swift
//
import SwiftUI
struct Timeline {
let id: Int
let name: String
let image: String
let post: String
let post_image: String
}
let timelines: [Timeline] = [
Timeline(id: 0, name: "Arupaka", image: "animal_arupaka", post: "This is post content", post_image: "ice_1"),
Timeline(id: 1, name: "Buta", image: "animal_buta", post: "This is post content", post_image: "ice_2"),
Timeline(id: 2, name: "Hamster", image: "animal_hamster", post: "This is post content", post_image: "flower"),
Timeline(id: 3, name: "Hiyoko", image: "animal_hiyoko", post: "This is post content", post_image: "moon"),
Timeline(id: 4, name: "Inu", image: "animal_inu", post: "This is post content", post_image: "animal_inu")
]
struct TimelineView: View {
let timelines: [Timeline]
var body: some View {
VStack() {
ForEach(self.timelines, id: \.id) { (timeline) in
VStack(spacing: 0) {
HStack {
Image(timeline.image)
.resizable()
.clipShape(Circle())
.overlay(
Circle().stroke(Color.white, lineWidth: 4))
.frame(width: 50, height: 50, alignment: .leading)
Text(timeline.name)
.fontWeight(.bold)
Spacer()
Image(systemName: "list.bullet")
}
.padding(.horizontal, 5)
Divider()
Image(timeline.post_image)
.resizable()
.scaledToFill()
.frame(width: UIScreen.main.bounds.width, height: UIScreen.main.bounds.width, alignment: .center)
.clipShape(Rectangle())
Divider()
Group {
Text("(timeline.name) ").fontWeight(.bold) +
Text(timeline.post)
}
.padding(.horizontal, 5)
.frame(width: UIScreen.main.bounds.width, alignment: .leading)
}
}
}
}
}
struct TimelineView_Previews: PreviewProvider {
static var previews: some View {
TimelineView(timelines: timelines)
}
}
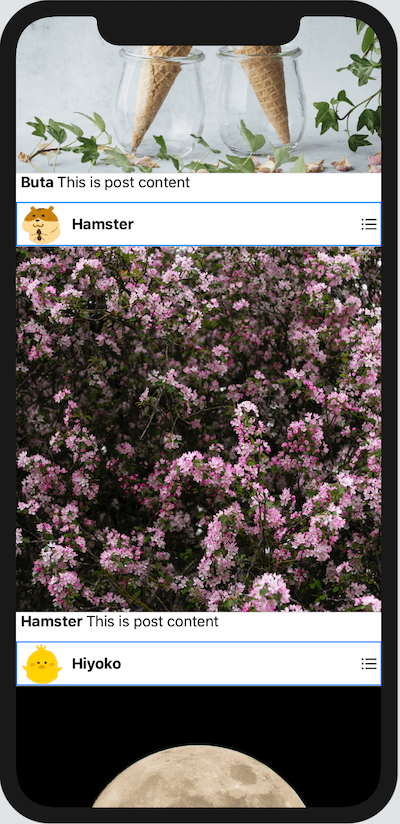
Build elements - ContentView.swift
Display StoryView
and TimelineView
with VStack
.
//
// ContentView.swift
//
import SwiftUI
struct ContentView: View {
init() {
UINavigationBar.appearance().titleTextAttributes = [.font : UIFont(name: "Georgia", size: 26)!]
}
var body: some View {
TabView {
NavigationView {
ScrollView(.vertical, showsIndicators: false) {
VStack {
StoryView(stories: stories)
Divider()
TimelineView(timelines: timelines)
}
}
.navigationBarTitle(Text("Instagram"), displayMode: .inline)
.navigationBarItems(
leading: IconView(systemName: "camera"),
trailing: HStack{
IconView(systemName: "tv")
IconView(systemName: "paperplane")
.padding(.leading, 10)
}
.padding(.bottom, 10)
)
}
.tabItem {
IconView(systemName: "house")
}
IconView(systemName: "magnifyingglass")
.tabItem {
IconView(systemName: "magnifyingglass")
}
IconView(systemName: "plus.app")
.tabItem {
IconView(systemName: "plus.app")
}
IconView(systemName: "heart")
.tabItem {
IconView(systemName: "heart")
}
IconView(systemName: "person")
.tabItem {
IconView(systemName: "person")
}
}
.edgesIgnoringSafeArea(.top) // Not needed after Xcode11.4
.accentColor(.black)
}
}
struct IconView: View {
var systemName: String
var body: some View {
Image(systemName: systemName)
.font(.title)
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
References
navigationbar - How can the background or the color in the navigation bar be changed? - Stack OverflowHow to render a gradient - a free SwiftUI by Example tutorial
Materials
かわいいフリー素材集 いらすとやBeautiful Free Images & Pictures | Unsplash