Switching Screens with TabView
Published by @SoNiceInfo at 6/24/2020
TabView
allows you to switch between multiple screens.
How to use TabView
TabView
is a feature that displays a tab at the bottom of the screen so that you can switch screens.
The View directly under TabView
is the content of each tab.
The tab function can be applied by using the tabItem
modifier for the View.TabView
complies with the View protocol, so View modifiers are available.
Describes the contents of a tab bar in tabItem
.
Note that the View that can be used in tabItem
is limited to Text and Image.
If you use something other than Text or Image, it will be an empty tabItem
.
//
// ContentView.swift
//
import SwiftUI
struct ContentView: View {
var body: some View {
TabView {
Image(systemName: "faceid")
.tabItem {
Image(systemName: "1.square.fill")
Text("faceid")
}
Image(systemName: "faceid")
.resizable()
.scaledToFit()
.frame(width: 200.0, height: 200.0)
.foregroundColor(.blue)
.tabItem {
Image(systemName: "2.square.fill")
Text("Second")
}
Image(systemName: "faceid")
.resizable()
.scaledToFit()
.font(.system(size: 18, weight: .ultraLight, design: .serif))
.frame(width: 200.0, height: 200.0)
.foregroundColor(.blue)
.tabItem {
Image(systemName: "3.square.fill")
Text("Third")
}
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
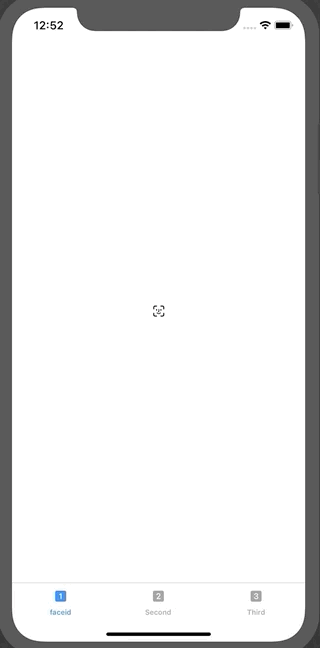
Change color of TabView
To change the color of the tab when it is selected, use .accentColor(.red )
.
To change the color of the tabs, you can't change the color of the tabs with the TabView modifier, so you need to change it on the UIKit side.
In the View initializer, specify UITabBar.appearance().backgroundColor
.
//
// ContentView.swift
//
import SwiftUI
struct ContentView: View {
init() {
UITabBar.appearance().backgroundColor = UIColor.blue
}
var body: some View {
TabView {
Image(systemName: "faceid")
.tabItem {
Image(systemName: "1.square.fill")
Text("faceid")
}
Image(systemName: "flame")
.tabItem {
Image(systemName: "2.square.fill")
Text("flame")
}
}.accentColor(.red)
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
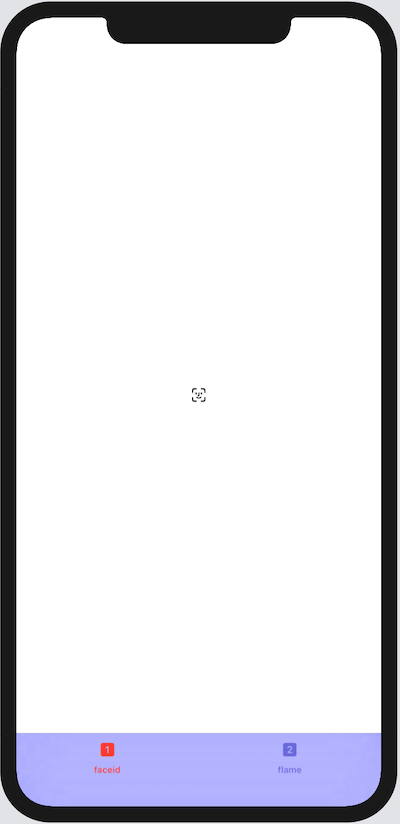