Define View
Published by @SoNiceInfo at 6/24/2020
You can define View
and use them anytime.
Views can be defined and called in a separate file.
About View
View
is very important in SwiftUI.
You can create a View
by describing the View
you want to display in the body, conforming to the View
protocol when defining the structure. By calling View
in View
, apps are made.
In fact, elements like Text
, Image
, and HStack
are all View
.
Creating View
You can define your own View
to combine the same code into one, which makes development and maintenance easier.
The basic type for creating a View
looks like this and can be called from another View
with ViewName()
.
struct ViewName: View {
var body: some View {
Text("This is custom View")
}
}
Example
Here is a View
called EmojiTile
. EmojiTile
is a square-like View
that uses the received string and colors to display.
Various modifiers are available in View
.
Here we are using padding
(margins) and cornerRadius
(rounded corners).
When the EmojiTile
is ready, call it in ContentView
.EmojiTile
is called vertically in VStack
.
//
// ContentView.swift
//
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
EmojiTile(text: "😀", color: .blue)
EmojiTile(text: "😉", color: .green)
EmojiTile(text: "😊", color: .purple)
EmojiTile(text: "😀", color: .blue)
}
}
}
struct EmojiTile: View {
var text: String
var color: Color
var body: some View {
Text(text)
.font(.custom("Emoji", size: 50))
.padding(50)
.background(color)
.cornerRadius(10)
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
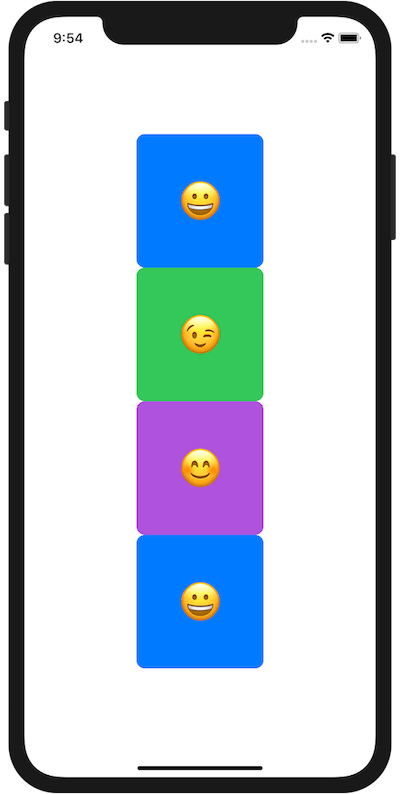
Separate View to a File
You can write your own View
into a separate file.
If you write everything in ContentView.swift
, you will not be able to continue to develop with it's readability.
I'll write EmojiTile
to a file called EmojiTile.swift
.
//
// ContentView.swift
//
import SwiftUI
struct ContentView: View {
var body: some View {
VStack {
EmojiTile(text: "😀", color: .blue)
EmojiTile(text: "😉", color: .green)
EmojiTile(text: "😊", color: .purple)
EmojiTile(text: "😀", color: .blue)
}
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
//
// EmojiTile.swift
//
import SwiftUI
struct EmojiTile: View {
var text: String
var color: Color
var body: some View {
Text(text)
.font(.custom("Emoji", size: 50))
.padding(50)
.background(color)
.cornerRadius(10)
}
}
struct EmojiTile_Previews: PreviewProvider {
static var previews: some View {
EmojiTile(text: "", color: .blue)
}
}
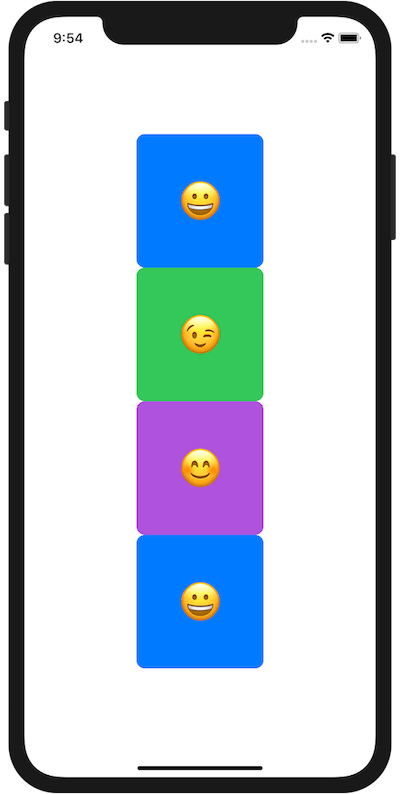