Display Map
Published by @SoNiceInfo at 6/24/2020
Use MapKit
to display the map.
iOS 14 Support
Only using Map(coordinateRegion: Binding<MKCoordinateRegion>)
, a map is displayed.
The result of this code is the same as the screenshot below.
import SwiftUI
import MapKit
struct ContentView: View {
@State private var region = MKCoordinateRegion(center: CLLocationCoordinate2D(latitude: 35.655164046, longitude: 139.740663704), span: MKCoordinateSpan(latitudeDelta: 0.1, longitudeDelta: 0.1))
var body: some View {
Map(coordinateRegion: $region)
.edgesIgnoringSafeArea(.all)
}
}
iOS 13 Support
You should make UIViewRepresentable
to make MKMapView be usable in SwiftUI
UIViewRepresentable
You can't display a map with the standard SwiftUI features.
You need to use MapKit to view the map.
Use UIViewRepresentable
to use MapKit in SwiftUI.UIViewRepresentable
is used to use UIKit in SwiftUI.
makeUIView
makeUIView
initializes and creates a UIView for UIKit.
It is called first and only once in the UIViewRepresentable structure.
When there is an update, updateUIView
is called.
In this page, I prepare MKMapView
of MapKit.
updateUIView
updateUIView
is called when there is an update on the View.
The initial position and scale must be set in updateUIView
to use MapKit.CLLocationCoordinate2D
is used to coordinate latitude and longitude with Representation. In this example, the latitude and longitude of Tokyo Tower are used.MKCoordinateSpan
represents a scale.
Set the latitude and longitude and scale of the map you want to set in MKCoordinateRegion
.
Finally, apply setRegion()
to MKMapViwe's uiView.
//
// ContentView.swift
//
import SwiftUI
import MapKit
struct ContentView: View {
var body: some View {
MapView()
.edgesIgnoringSafeArea(.all)
}
}
struct MapView: UIViewRepresentable {
func makeUIView(context: Context) -> MKMapView {
MKMapView(frame: .zero)
}
func updateUIView(_ uiView: MKMapView, context: Context) {
let coordinate = CLLocationCoordinate2D(
latitude: 35.655164046, longitude: 139.740663704)
let span = MKCoordinateSpan(latitudeDelta: 0.1, longitudeDelta: 0.1)
let region = MKCoordinateRegion(center: coordinate, span: span)
uiView.setRegion(region, animated: true)
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
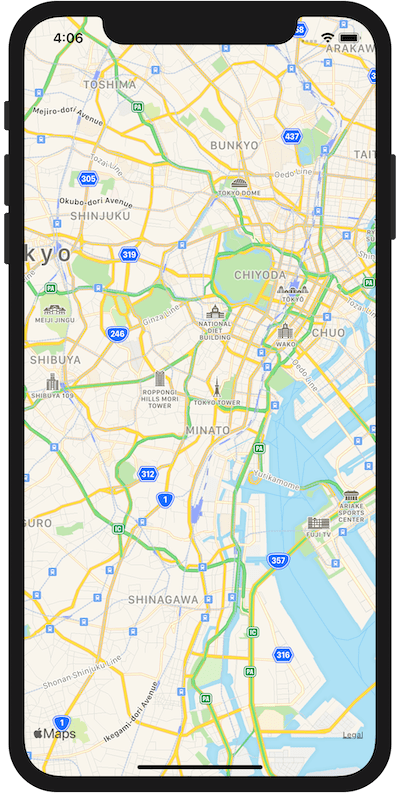
References
Creating and Combining Views — SwiftUI Tutorials | Apple Developer DocumentationMKMapView - MapKit | Apple Developer Documentation